The world’s first public Angular conference kicked off today in Salt Lake City and I was excited to attend with Wintellect. Our company has been using Angular along with other tools like TypeScript to build enterprise apps scaled across large development teams for a year now, and were able to bring most of the team who has been hands-on with this toolset to the conference. It was a great day interacting with a massive community who are building apps in every vertical. In this post I want to share some of the highlights from the conference.
The first thing they discussed was authorization and activation with a user profile. They need to get the user profile information into the app and reduce the overhead of doing this with every call. Their solution was to render the information on the server, pull it into Angular using the copy service and declare it as a constant to reference anywhere. They then implemented conditional feature loaded by extended the HTML with attributes that essentially mark up what features can be turned on or off. If the user doesn’t have the feature, that DOM Node is removed which takes it a step beyond just “show” and “hide.”
The next section was about code organization. I was happy to see we independently came up with the same best practices their team was using – things like preferring composition over inheritance, using mix-ins but only sparingly (using Angular’s extend), but most importantly favoring Angular services. This is where our app has the most mileage for reuse and simplifying controllers, by taking well-defined actions or processes and placing them in services that can be shared across controllers.
They next mentioned how to use services to extend the Angular built-in services. Again, this mirrored a solution we came up with. We extended the $http service, they chose to do it with the $resource service. They exposed a fluent interface to configure the service and extended it to return actual objects and not just $http promises. We provide services that implement a base class to wrap $http that does custom error handling among other things, then returns first class entities based on the nature of the request, like musicService.getSong(“lullabye”).then(function(song) { song.sing(); }
The slides from the presentation are shared here.
Promises address this through a common API that modules can agree. They don’t just replace callbacks, but create rich functionality around them. A great example is the example that you can run three processes in parallel and use $q.all to run only when the three other promises completed.
Christian Lilly suggested several steps to getting familiar with the promises ($q) API in Angular. Step one? Consume existing promises by using services like $http. Step two? Start using $resource. Step three is to use the $routeProvider and familiarize yourself with $route: resolve that enables deferred rendering until a promise is completed. The final step is to recognize that Angular no longer unwraps promises in views. It was too much magic so the wizards destroyed the scrolls. Step five was to start creating your own promises using the $q service. Step six is to use third-party libraries that take advantage of promises such as Angular.UI. Step seven is to create a lazy promise by wrapping a promise in a service. Step eight is to learn about interceptors in the $http lifecycle.
A great way to learn about directives is to read the Angular source code to see how they implemented their own directives.
Use directives when you want a reusable HTML component, a reusable HTML behavior, you want to wrap a jQuery plug-in and almost any time you need to interface with the DOM.
He recommended the practice of placing directives in their own module. This allows you to unit test them independent of the rest of the application (of course you need to pull it in as a dependency for your main app). Another suggestion is to include a script tag of type text/ng-template with an id equal to the URL of the directive. This will pull the directive in once and cache it.
He shared various ways to build behaviors, custom HTML and even widgets using directives and covered scope isolation as well. He noted how you can use attributes to pass data that are outside of the $digest loop and don’t require creating a new $scope. Use $eval to pull in the attribute values and $set to set a new value on the attribute.
Link is used for the initial pass of rendering the directive. If you need to manipulate DOM after link time or reuse a template multiple times, you need a compile function.
Great description of transclusion: it is a “picture frame” for content. You either sell the frame with your picture inside, or you sell the frame and allow consumers to put their own content inside. Slides from the talk are posted here.
On that the morning session wrapped. So far this has been a terrific conference!
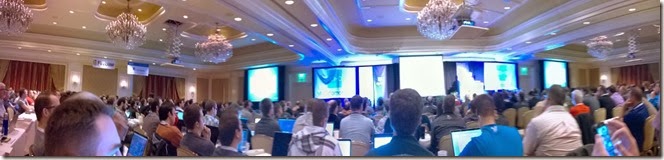
Keynote – History of Angular
The keynote was kicked off by creator and original contributor Miško Hevery and Brad Green. They started by sharing some of the history for Angular. I’ve been sharing that I feel one of the powerful features of angular is the developer/designer workflow. It enables the XAML-style workflow many C# developers are used to in pure HTML scenarios. This was reinforced in the keynote when they mentioned the original Angular was written to make things easier for designers and non-programmers rather than developers. It of course grew from there over time, but the initial view was a sort of advanced spreadsheet for the cloud The first test of Angular was internal at Google. An existing application had been built over 6 months and contained 17,000 lines of code. Miško offered to take this on and in weeks was able to recreate the app using Angular and simplified it to 1,500 LOC. The benefits they found mirror what Wintellect has experience: faster production of code, improved quality, easier readability, better designer/developer workflow and testable, modular code that scales across teams. To give you an idea of how much the framework has grown, there are now over 600 contributors and the project receives 10 – 15 pull requests per day. They also shared their keynote slides.Future of Angular 2.0
The goal for Angular 1.0 was to provide support for the browsers of yesterday. The upcoming 2.0 release will be focused on the browsers of tomorrow. This includes several features that were covered at a high level in the keynote, including:- A new benchmarking framework
- Lazy loading for dependency inject and support for ECMAScript 6
- Faster change detection, taking advantage of Object.observe when possible
- Better poly-fills
- Improved support for mobile
- Built-in offline support
- Active animations
- Reduction of initial load time
- Faster performance
- The team will also build a flagship app for mobile
- Reduce API complexity
- Simplify directives
- Dependency injection
- More declarative code with type and data annotations
- Support for ECMAScript 6
- Classes
- Modules
- Annotations
- Support for Standard widget libraries
- Shadow DOM
- DOM Mutation observers
- Object.observe
- Improved debugging
- Interoperability with zone.js that will allow elimination of need for $digest and $apply
- Interoperability with diary.js
AngularJS in 20 Minutes
Dan Wahlin gave a great overview of what Angular is in 20 minutes. Kudos to him for building a tiny app live in front of the group. He focused on the essentials: data-binding, directives, filters, modules, controllers, routes, animations, etc. In just a few minutes he demonstrated the power of Angular by creating an app that held a list of customers in memory and provided a search filter to select customers that matched text input by the user. It is a testament to how powerful and flexible the framework is that he was able to wire this up without writing a line of code in the beginning.Building Massive Angular Apps: DoubleClick
The next panel discussed what it took to build a massive application using Angular. I was very intrigued by this because we are also currently working on a huge enterprise app. The team indicated their app was on the framework they believe the longest (they started very early) and possibly the largest (right now it looks like ours might be larger). The app is the DoubleClick Campaign Manager (DCM) and is used by publishers to upload and track their ad campaigns. The project started using Angular in November of 2010 (we started in early 2013). They have over 20 JavaScript engineers, 71,000 lines of JavaScript, 133,000 lines of unit test code, 26,000 lines of markup, 129 controllers, 137 directives, and 59 services. By just the service and controller app, one of our projects is actually larger than this.The first thing they discussed was authorization and activation with a user profile. They need to get the user profile information into the app and reduce the overhead of doing this with every call. Their solution was to render the information on the server, pull it into Angular using the copy service and declare it as a constant to reference anywhere. They then implemented conditional feature loaded by extended the HTML with attributes that essentially mark up what features can be turned on or off. If the user doesn’t have the feature, that DOM Node is removed which takes it a step beyond just “show” and “hide.”
The next section was about code organization. I was happy to see we independently came up with the same best practices their team was using – things like preferring composition over inheritance, using mix-ins but only sparingly (using Angular’s extend), but most importantly favoring Angular services. This is where our app has the most mileage for reuse and simplifying controllers, by taking well-defined actions or processes and placing them in services that can be shared across controllers.
They next mentioned how to use services to extend the Angular built-in services. Again, this mirrored a solution we came up with. We extended the $http service, they chose to do it with the $resource service. They exposed a fluent interface to configure the service and extended it to return actual objects and not just $http promises. We provide services that implement a base class to wrap $http that does custom error handling among other things, then returns first class entities based on the nature of the request, like musicService.getSong(“lullabye”).then(function(song) { song.sing(); }
The slides from the presentation are shared here.
Promises
The next talk covered promises. What was unique about this talk was that he covered how promises aren’t just for asynchronous processes, but really designed to handle uncertainty and wrap your brain around unpredictable processes. He mentioned how callbacks were the initial approach to asynchronous tasks that don’t block I/O, but fall short because they are complex and require heavy nesting.Promises address this through a common API that modules can agree. They don’t just replace callbacks, but create rich functionality around them. A great example is the example that you can run three processes in parallel and use $q.all to run only when the three other promises completed.
Christian Lilly suggested several steps to getting familiar with the promises ($q) API in Angular. Step one? Consume existing promises by using services like $http. Step two? Start using $resource. Step three is to use the $routeProvider and familiarize yourself with $route: resolve that enables deferred rendering until a promise is completed. The final step is to recognize that Angular no longer unwraps promises in views. It was too much magic so the wizards destroyed the scrolls. Step five was to start creating your own promises using the $q service. Step six is to use third-party libraries that take advantage of promises such as Angular.UI. Step seven is to create a lazy promise by wrapping a promise in a service. Step eight is to learn about interceptors in the $http lifecycle.
Advanced Custom Directives
Dave Smith gave a very informative and entertaining talk about custom directives. A few tip he provided:- Use a unique prefix (don’t clash with others and make it easier for readers to identify)
- DON’T use ng-
- Common convention is two letters – that’s 1,296 possibilities
A great way to learn about directives is to read the Angular source code to see how they implemented their own directives.
Use directives when you want a reusable HTML component, a reusable HTML behavior, you want to wrap a jQuery plug-in and almost any time you need to interface with the DOM.
He recommended the practice of placing directives in their own module. This allows you to unit test them independent of the rest of the application (of course you need to pull it in as a dependency for your main app). Another suggestion is to include a script tag of type text/ng-template with an id equal to the URL of the directive. This will pull the directive in once and cache it.
He shared various ways to build behaviors, custom HTML and even widgets using directives and covered scope isolation as well. He noted how you can use attributes to pass data that are outside of the $digest loop and don’t require creating a new $scope. Use $eval to pull in the attribute values and $set to set a new value on the attribute.
Link is used for the initial pass of rendering the directive. If you need to manipulate DOM after link time or reuse a template multiple times, you need a compile function.
Great description of transclusion: it is a “picture frame” for content. You either sell the frame with your picture inside, or you sell the frame and allow consumers to put their own content inside. Slides from the talk are posted here.
On that the morning session wrapped. So far this has been a terrific conference!